How to integrate with ATS (Hire API)
How to develop an integration with Applicant Tracking Systems (ATS)
Bob's Hire API simplifies the people onboarding process by seamlessly integrating an Applicant Tracking System (ATS) with Bob, effortlessly adding new hires as employees in Bob. This integration eliminates the need for manual export of hired candidates from ATS systems, as they are automatically created as employees in Bob.
Bob’s Hire API workflow:
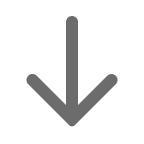
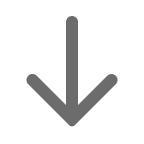
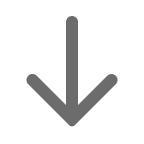
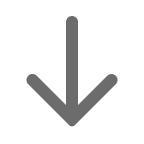
The following sections detail how to implement the integration on the ATS side and are aimed at ATS developers who want to integrate with Bob and push new candidates as Hires in Bob.
Note: This is the developer’s guide for implementing a new Hire API integration for an ATS. If you want to set up an existing integration, refer to How to set up Hire API integration in Bob ↗.
Endpoint URL and Authorization Header
The Bob Hire API endpoint URL and authentication details are dynamically generated when the user installs the integration in Bob.
During installation, the user selects an authentication method. Based on the chosen method, the endpoint URL and authentication details are generated on-the-fly. The Hire API has a base URL, and the authentication method is appended to this URL as a service user ID or a token id. These details are essential for securely accessing and interacting with the Bob Hire API, allowing users to send and receive data as part of the integration process.
To learn more, see Endpoint URL and Authorization header.
First, let's go into the steps.
Implementing the Integration
Implementing the integration consists of the following development steps:
- Step 1: Setup.
In order to integrate with Bob's Hire API, you need to call the endpoint when you have a new Hire in the ATS. When developing the integration setup on the ATS side, you should obtain the endpoint's URL and authentication details which are dynamically generated in Bob. - Step 2: Prepare payload.
Develop the code that creates the new candidate’s payload. - Step 3: Call the Hire API.
Develop the code that calls the Hire API endpoint with the candidate’s details when there is a new hire in the ATS. - Step 4: Error handling.
Test the integration and handle errors.
Step 1: Setup
In this step you should develop a user interface to obtain the endpoint URL and the authentication details from Bob during the integration setup.
The following details are required in order to call the Hire API integration:
- Endpoint URL: The Bob Hire API endpoint URL is generated when the user activates the integration in Bob.You should provide the user a way to copy and paste it into your ATS integration configuration, so you can use it in the HTTP POST requests. To learn more, see Endpoint URL.
- Authentication details: The authentication details (service user ID or Token ID) are generated when the user activates the integration in Bob. You should provide the user a way to copy and paste the authentication details into your ATS integration configuration. To learn more, see Authorization header.
Step 2: Prepare payload
In this step you should prepare the payload that you will send to the POST request. The payload will contain the candidate’s details.
As best practice, we advise on using the field names as they appear in the default Hire API field mapping. This will ensure your candidate details will be automatically propagated into the correct fields in Bob.
Tip: If you need to send fields which are not part of the basic Hire API field mapping, you can use custom mapping. To learn more, see Including custom fields in the payload.
Payload format
The payload with the candidate details in the request body should be a JSON file.
Ensure that the payload includes all relevant information about the candidate. There are no mandatory fields.
The payload can include any of the following fields:
- Employee fields that are mapped automatically by Bob. Make sure you send the correct field Ids in the payload, as listed in the Candidate fields.
- Document fields (for attachments). To learn how to send documents, see Document fields.
- Custom Fields. To learn more about sending custom fields, see Using custom fields in the payload.How a Hire API integration works
Including custom fields in the payload
You may have custom fields which are not part of the default fields that are included in the Hire API, and you want to propagate them into fields in Bob.
If you want to pass custom fields, you need to instruct the end-user to manually map these fields after the first time they pass a new hire through the integration. Custom fields are not part of the default data mapping of the Hire API.
Here’s what you should do to include custom fields:
- Include all the fields in the payload: All fields should be included in the payload, so they will be recognized as part of the field mapping. To learn more, see Mapping custom fields.
- Instruct users: As part of your setup instructions, inform users to follow Bob’s Hire API setup instructions for manually mapping the fields after receiving a new hire in Bob for the first time. This ensures that the custom fields will be recognized and properly mapped within Bob.
- Include mapping details: Your instructions should include details of the fields that should be mapped to the equivalent fields in Bob.
Payload example
An example of a candidate’s name with an attachment of a CV file:
{
"email": "[email protected]",
"firstName": "Joe",
"lastName": "Doe",
"documents": [
{
"name": "CV.pdf",
"url": "https://www.bellevue.edu/student-support/career-services/pdfs/resume-samples.pdf"
},
{
"name": "cover_letter.pdf",
"url": "http://skcnedu.in/uploads/files/document43.pdf"
}
]
}
For more examples, see Payload Examples.
Step 3: Call the Hire API
In this step you should develop the code that actually calls the Hire API endpoint and sends the payload with the candidate details.
Calling the Hire API can be done in two ways:
- Automatically (Using a Webhook): Create a webhook event in the ATS which sends the POST request to the Hire API endpoint whenever a new candidate is hired.
- Manually using a user action in the UI: Provide the user a way to manually push the new candidate from the ATS into Bob using a button, and call the Hire API.
Endpoint URL
This is the automatically generated endpoint URL to which you should send your HTTPS POST request with the new candidate details.
The base URL for the API endpoint is:
https://app.hibob.com/api/integrations/ats/hire
To this base URL, Bob appends the details as follows:
- Service user authentication:
https://app.hibob.com/api/integrations/ats/hire/<integration id>
The integration id is generated automatically when setting up the service user for this integration. - Token authentication:
https://app.hibob.com/api/integrations/ats/hire?token=<token id>
The token id is generated automatically when setting up the token id for this integration.
In addition, Bob generates a secret key that should be used in the Authorization header.
Authorization header
Each time you call the Hire API with an HTTP POST request, it must include the HTTP Authorization header with the API authentication details.
Bob supports two authentication methods which you can use for your integration:
- Service user
When your authentication method is based on a service user, you need to include the service user credentials encoded in base64 in the request's authorization header. In order to create the authorization header, you will need to obtain the service user id and token from Bob when setting up the integration.
To learn more about using service users to access the Bob API, see API Service users. - Token
When your authentication method is based on a token, use the secret key generated by Bob in the integration setup stage, to generate a signature for your request's authorization header. This involves creating a hash or encoded signature that validates your request's authenticity.
To learn more about using the token to access the Hire API, see How to use the Token in the authentication header.
How to use the Token in the authentication header
When your authentication method is based on a token, you need to sign your request using a secret key and include the calculated signature in the request header.
Token based authentication requires you to include the following HTTP headers in a request:
- X-Bob-HireAPI-Timestamp - epochValue (utc timestamp) in seconds. It must be at most 5 minutes from the current time.
- X-Bob-HireAPI-Signature - HMAC-SHA256 signature of the payload. To create the signature you will need to use the epochValue, and the Secret Key obtained from HiBob.
How to compute a signature
The signature is computed as follows:
message = epochValue+requestBody
signature\* = HMAC-SHA256(message,SecretKey)
Where:
- epochValue: The value received in X-Bob-HireAPI-Timestamp header.
- requestBody: The request body that will be sent to HiBob.
- SecretKey: The secret obtained when setting-up the HireAPI integration.
Example:
epochValue = 1676691263
requestBody = { “email”: “[email protected]”, “firstName: “Joe”, “lastName”: “Doe” }
SecretKey = 4BBE2E0E43D0FC230870E760E11FE19789AA535A
How to compute:
- Store epochValue in request header X-Bob-HireAPI-Timestamp.
- Prepend requestBody with epochValue resulting in following:
message = 1676691263{ “email”: “[email protected]”, “firstName: “Joe”, “lastName”: “Doe”}
signature* = Calculate HMAC-SHA256 using message from previous step
HMAC_SHA256(signature, SecretKey)
- Store signature in request header X-Bob-HireAPI-Signature
Notes:
- You can use https://codebeautify.org/hmac-generator for debugging signature-related problems.
- There must be no character between the epochValue and the requestBody. The above + sign represents concatenation in the above signature.
Using the URL as a Webhook
Webhooks are a powerful tool for enabling real-time communication and event-driven interactions between systems.
When using the Hire API endpoint, you'll send an HTTPS POST request to Bob's endpoint, including candidate details.
When developing the integration on the ATS side, you may consider implementing it using a webhook event in your ATS system, which means you will call the Hire API as a webhook endpoint, whenever a new hire event occurs in your system.
HTTP POST request example
POST /api/integrations/ats/hire/<service user/token> HTTP/1.1
Host: app.hibob.com
Content-Type: application/json
Authorization: ••••••
Content-Length: 162
{
"email": "[email protected]",
"firstName": "Joe",
"lastName": "Doe",
"recruiterEmails": [
"[email protected]",
"[email protected]"
]
}
Step 4: Error Handling
Your implementation should deal with scenarios where things don't work as expected:
- deal with a scenario where the endpoint URL is unreachable or returns errors.
- Implement retry mechanisms for failed deliveries to ensure the reliability and resilience of the integration.
- When anything goes wrong, instruct end-users to check the payload in Bob's UI. Bob stores the payload that is received as-is, so users can track the events that were received.
Hire API field mapping
The fields listed below will be automatically mapped when sending them in the new candidate’s details payload.
How field mapping works:
- None of the fields are mandatory.
- Manual field mapping will be enabled once the first Hire event is triggered.
- By default, Bob automatically matches out-of-the-box fields that are sent with the field IDs listed below.
- Bob will only parse exact matches.
- If the payload includes fields that are not mapped with Bob’s default field mapping, you will need to tell the user to use the manual field mapping when setting up the integration.
- Using the data-mapping component in the Hire API connections page in Bob the user can customize the field mapping and map fields that are in the payload to fields in Bob.
Candidate's fields
Field | Type | Description |
---|---|---|
text | The employee email | |
firstName | text | The employee first name |
lastName | text | The employee last name |
recruiterEmails | List of text | The list of recruiter emails that will receive the bob new hire notification email |
work/title | text | The employee work title |
work/department | text | The employee work department |
work/site | text | The employee work site |
work/reportsTo | text | The name or email of the employee manager |
work/startDate | date | The employee work start date |
financial/employmentContract | text | The employee employment contract |
financial/employmentType | text | The employee employment type |
financial/payPeriod | text | The employee pay period |
financial/salary | number | The employee salary |
financial/salaryCurrency | text | The employee salary currency |
about/privatePhone | text | The employee personal phone |
about/mobilePhone | text | The employee personal mobile |
about/linkedinProfile | text | The employee LinkedIn profile URL |
about/facebookProfile | text | The employee Facebook profile URL |
about/twitterProfile | text | The employee Twitter profile URL |
Documents fields
For Bob to receive documents from the ATS you will need to include the following fields:
Field | Type | Description |
---|---|---|
documents/name | text | The name for the document that will be attached to the employee in Bob. Be sure to include the correct file extension, as the system uses it to process and attach the document. |
documents/url | text | The URL of the employee document (must be unsecured) |
Note: Documents that are attached to the request are automatically pulled from the ATS and saved in the employee's Docs > Confidential docs folder in Bob.
See the below example:
"documents": [
{
"name": "CV.pdf",
"url": "https://www.bellevue.edu/student-support/career-services/pdfs/resume-samples.pdf"
},
{
"name": "cover_letter.pdf",
"url": "http://skcnedu.in/uploads/files/document43.pdf"
}
]
Mapping custom fields
In addition to the default fields which are included in the Hire API, Bob can accept custom fields. Custom fields must include a unique identifier in the ATS (Applicant Tracking System) to ensure proper integration and field mapping.
Each custom field must contain the following properties:
- ID: A unique identifier of the field in the ATS. Ensure this field has a unique ID to maintain data integrity.
- Display Name: The name that will be shown in Bob’s UI during field mapping
- Field Type: The type of the field, which can be one of the following: Text, Number, Date, Currency, Timestamp, Boolean, Multilist, Table
- Field Value: The value that matches the field type.
Field Mapping
Custom fields should be mapped manually in Bob’s system after the initial setup and the first time a new hire is passed through the integration.
During this process, The user will map the custom fields based on their unique identifiers to corresponding fields within Bob’s UI. This ensures that all custom data is accurately integrated and available within Bob.
Ensure that the custom fields are mapped accurately during the integration setup to prevent any data mismatches or integration errors.
Custom fields examples
See the following examples of custom fields that can be included in your request:
Text:
"customFields": [
{
"id": "text_field_1”,
"displayName": "moto",
"fieldType": "text",
"value": "Blue, Yellow and Red"
}
]
Number
"customFields": [
{
"id": "number_field_1",
"displayName": "Favorite Number",
"fieldType": "number",
"value": 123.56
}
]
Date
"customFields": [
{
"id": "date_field_1",
"displayName": "Birthday",
"fieldType": "date",
"value": "2019-11-15"
}
]
Currency
{
"id": "currency_field_1",
"displayName": "poker - best win",
"fieldType": "currency",
"value": {
"value": 12.33,
"currency": "USD"
}
}
Timestamp
{
"id": "timestamp_field_1",
"displayName": "Favorite Moment",
"fieldType": "timestamp",
"value": "2019-10-15T22:49:14Z"
}
Boolean
"customFields": [
{
"id": "boolean_field_1",
"displayName": "Boolean Field",
"fieldType": "boolean",
“value": true
}
]
Multilist
"customFields": [
{
"id": "multi_list_field_1",
"displayName": "Favorite Sports",
"fieldType": "multilist",
"values": [
"Football",
"Handball",
"value3"
]
}
]
Table
"customFields": [
{
"id": "table_type_list_field_1",
"displayName": "Table Type Field",
"fieldType": "tableType",
"values": [
[
{
"id": "c11",
"displayName": "C 11",
"fieldType": "text",
"value": "1"
},
{
"id": "c12",
"displayName": "C 12",
"fieldType": "text",
"value": "2"
}
],
[
{
"id": "c21",
"displayName": "C 21",
"fieldType": "text",
"value": "3"
},
{
"id": "c22",
"displayName": "C 22",
"fieldType": "text",
"value": "4”
}
]
]
}
]
Linking with fields in Bob (e.g. position id)
"customFields": [
{
"id": "number_field_3",
"displayName": "Position ATS",
"fieldType": "text",
"value": "P-1678797"
}
]
Payload examples
The following is a list of examples of different types of payloads.
Basic, with just email, firstName, lastName and recruiteEmails fields
{
"email": "[email protected]",
"firstName": "Joe",
"lastName": "Doe",
"recruiterEmails": [
"[email protected]",
"[email protected]"
]
}
Example with work, financial and about categories populated along with documents
{
"email": "[email protected]",
"firstName": "Joe",
"lastName": "Doe",
"recruiterEmails": [
"[email protected]",
"[email protected]"
],
"work": {
"title": "Account Manager",
"department": "Distribution",
"site": "Cluj-Napoca",
"reportsTo": "[email protected]",
"startDate": "2019-10-21"
},
"financial": {
"employmentContract": "Full Time",
"employmentType": "Permanent",
"payPeriod": "Annual",
"salary": 12500.5,
"salaryCurrency": "USD"
},
"about": {
"privatePhone": "021343536",
"mobilePhone": "021444555",
"linkedinProfile": "http://linkedin.com/in/joe-doe",
"facebookProfile": "http://facebook.com/joedoe2",
"twitterProfile": "http://twitter.com/jd2"
},
"documents": [
{
"name": "CV.pdf",
"url": "https://www.bellevue.edu/student-support/career-services/pdfs/resume-samples.pdf"
},
{
"name": "cover_letter.pdf",
"url": "http://skcnedu.in/uploads/files/document43.pdf"
}
]
}
An example of custom fields
{
"email": "[email protected]",
"firstName": "Joe",
"lastName": "Doe",
"recruiterEmails": [
"[email protected]",
"[email protected]"
],
"work": {
"title": "Account Manager",
"department": "Distribution",
"site": "Cluj-Napoca",
"reportsTo": "[email protected]",
"startDate": "2019-10-21"
},
"financial": {
"employmentContract": "Full Time",
"employmentType": "Permanent",
"payPeriod": "Annual",
"salary": 12500.5,
"salaryCurrency": "USD"
},
"about": {
"privatePhone": "021343536",
"mobilePhone": "021444555",
"linkedinProfile": "<http://linkedin.com/in/joe-doe">,
"facebookProfile": "<http://facebook.com/joedoe2">,
"twitterProfile": "<http://twitter.com/jd2">
},
"documents": [
{
"name": "CV.pdf",
"url": "https://www.bellevue.edu/student-support/career-services/pdfs/resume-samples.pdf"
},
{
"name": "cover_letter.pdf",
"url": "http://skcnedu.in/uploads/files/document43.pdf"
}
],
"customFields": [
{
"id": "text_field_1",
"displayName": "moto",
"fieldType": "text",
“value": "Blue, Yellow and Red"
},
{
"id": "number_field_1",
"displayName": "Favorite Number",
"fieldType": "number",
"value": 123.56
}
]
}
Updated 18 days ago